How to check if an object is empty in javascript
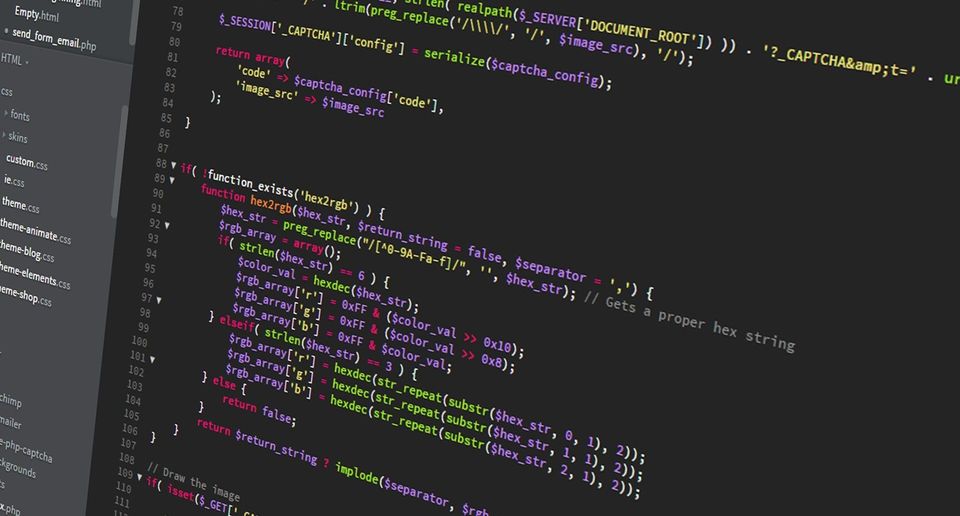
There are a few ways to check if an object is empty in Javascript.
But I care about performance and readability.
The fastest way is to use a for...in
loop.
You could use the below code snippet as a utility function.
function isEmpty(obj) {
for(let key in obj) {
if(obj.hasOwnProperty(key))
return false;
}
return true;
}
My favorite way for readability reasons and it's still high performing is using Object.keys
.
function isEmpty(obj) {
return Object.keys(obj).length === 0
}
Note: Object.keys
is faster than using Object.entries
.
Resources
for...in
The for...in statement iterates over all enumerable properties of an object that are keyed by strings (ignoring ones keyed by Symbols), including inherited enumerable properties.

Object.keys()
The Object.keys() method returns an array of a given object’s own enumerable property names, iterated in the same order that a normal loop would.
